OSM Viz
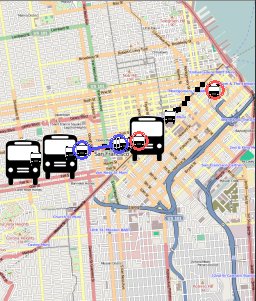
Some buses in San Francisco.
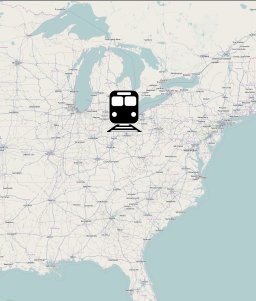
All aboard!
An OpenStreetMap Visualization Toolkit for Python
OSMViz is a small set of Python tools for retrieving and using OpenStreetMap (OSM) images (actually, Mapnik images served by Slippy Map). Its original purpose was to draw a bunch of things moving around on the map, which has been somewhat generalized and expanded.
With OSMViz you can:
- Grab appropriate rendered OSM tiles from an OSM server of your choosing
- Patch tiles together into a bigger map
- Easily animate stuff on that map (requires pygame)
A note about OpenStreetMap maps
If you choose to pull tiles from the openstreetmap.org server, then there are some things you should know:
- These tiles have a usage policy, available here.
- These tiles are (c) OpenStreetMap and contributors, CC-BY-SA
Using OSM Viz
The code below will show a train running across the USA. Choo choo!
from osmviz.animation import TrackingViz, Simulation
# Define begin/end points, duration, and icon for our train
start_lat,start_lon = (45.77,-68.65) # Northeast
end_lat,end_lon = (30.05,-118.25) # Southwest
begin_time, end_time = 0, 60 # In 60 seconds!
image_f = "images/train.png"
# Define bounds for the train and zoom level, how much map do we show?
bound_ne_lat,bound_ne_lon = (46,-68.5)
bound_sw_lat,bound_sw_lon = (30,-119)
zoom = 6 # OSM zoom level
# Define an interpolater to create animation points
def locAtTime(t):
if t < 0: return start_lat,start_lon
if t > 60: return end_lat,end_lon
frac = t/60.0
interp_lat = start_lat + frac * (end_lat-start_lat)
interp_lon = start_lon + frac * (end_lon-start_lon)
return interp_lat,interp_lon
# Create a TrackingViz
viz = TrackingViz("Continental Espresso", image_f, locAtTime,
(begin_time,end_time),
(bound_sw_lat,bound_ne_lat,bound_sw_lon,bound_ne_lon),
1) # Drawing order
# Add our TrackingViz to a Simulation and then run the simulation
sim = Simulation([viz,],[],0) # ([actor vizs], [scene vizs], initial time)
sim.run(speed=1,refresh_rate=0.1,osmzoom=zoom)
While the animation is running, you can control it as follows:
- Mousing over the icon displays its label ("Continental Espresso")
- Up/down arrows increase/decrease the speed of simulation (can go backwards in time)
- Left/right arrows set simulation to begin/end of the time window
- Space bar sets the speed to zero
- Escape key exits.
To run it yourself you need to have an existing "images/train.png" as well as an empty "maptiles/" directory. These already exist in the "test" directory in the source download; in fact one of the included tests is to show the same train.
Requirements
- Python (tested using Python 2.5.x)
- Animations require Pygame (tested using Pygame 1.9.1)
- Image construction requires PIL (tested using PIL 1.1.6)